This page requires JavaScript.
Please turn on JavaScript in your browser and refresh the page to view its content.

Fix HTML Video Autoplay Not Working
To optimise pages on this blog I recently replaced the animated GIFs with auto-playing videos. It required a couple tries to get it right, so here’s how it works.
The video below won’t autoplay.
To fix this we add the muted attribute, this will disable the audio, making the video less intrusive.
However the video above still won’t autoplay on iOS Safari, Safari requires us to add the playsinline attribute.
We can try to hack around these autoplay limitations with some JavaScript, but that will only work if the JavaScript is run as a result of a user action.
The code below won’t work, it will throw a warning in the developer console.
The play action below will work because the code runs as a result of a user action.
Press Play to play the first video on the page.
I share web dev tips on Twitter, if you found this interesting and want to learn more, follow me there
Or join my newsletter
Busy doing the newsletter subscribing.
Something went wrong, can you give it another go?
At PQINA I design and build highly polished web components.
Make sure to check out FilePond a free file upload component, and Pintura an image editor that works on every device.
Related articles
Typescript interface merging and extending modules, styling password fields to get better dots, how to prevent scrolling the page on ios safari 15.
Autoplay HTML5 video on iOS, android and desktop

Learn how to create a more accessible, auto-playing HTML5 video which works great as a replacement for animated GIFs and component background.
Before I jump into the code, let’s first look at the reasons for using HTML5 video as a replacement for animated GIFs:
Animated GIFs don’t compress very well. They have very large file sizes and the quality takes a massive hit as soon as we try to compress them.
.mp4 files (the file format we’ll be using for our videos), compress much better and maintain their quality. Here’s an example:
Comparison of a 13 second animated GIF and MP4
- Savings: 574%
That’s a huge saving! Unfortunately, it does mean more work on our part to set up the markup needed to create an auto-playing HTML5 video.
It’s also worth noting, prior to iOS10 auto-playing HTML5 video wasn’t possible on iOS devices. But that changed when Apple implemented the playsinline attribute (which needs to be added to our element to autoplay on iOS).
Setting up the markup
With the intro covered, let’s jump into the markup needed for our videos.
<video class="m-video__video js-video-video" poster="/img/showreel.jpg" autoplay loop muted playsinline> <source src="/video/showreel.mp4" type="video/mp4"> </video>
Ok, let’s take a look at what all of these attributes are for:
- poster - an image that will be shown whilst the video is loading
- autoplay - this will cause the video to autoplay as soon as it’s loaded
- loop - makes the video start again when it’s finished
- muted - prevents any audio from playing (a video must be muted or have no audio for it to autoplay on iOS)
- playsinline - this tells iOS devices to play videos inline (so the user doesn’t have to interact with it to make it play)
Play/pause button markup
To make our autoplaying videos more inclusive, we’re going to add play/pause buttons, so users can pause the video if they don’t want it to play.
There are many reasons someone might want to pause a video; ranging from saving battery life to suffering from vestibular disorders .
This can cause someones vestibular system to struggle to make sense of what is happening, resulting in loss of balance, vertigo, migraines, nausea, and hearing loss.
With that covered, let’s have a look at the markup:
<button class="a-button m-video__button js-video-play-button" aria-pressed="true" aria-label="Play video" type="button"> <svg class="a-icon a-button__icon" viewBox="0 0 16 16" xmlns="http://www.w3.org/2000/svg"> <title>Play video</title> <path d="M5.85355339,0.853553391 L12.2928932,7.29289322 C12.6834175,7.68341751 12.6834175,8.31658249 12.2928932,8.70710678 L5.85355339,15.1464466 C5.65829124,15.3417088 5.34170876,15.3417088 5.14644661,15.1464466 C5.05267842,15.0526784 5,14.9255015 5,14.7928932 L5,1.20710678 C5,0.930964406 5.22385763,0.707106781 5.5,0.707106781 C5.63260824,0.707106781 5.7597852,0.759785201 5.85355339,0.853553391 Z"></path> </svg> </button> <button class="a-button m-video__button js-video-pause-button" aria-pressed="false" aria-label="Pause video" type="button"> <svg class="a-icon a-button__icon" viewBox="0 0 16 16" xmlns="http://www.w3.org/2000/svg"> <title>Pause video</title> <rect x="4" y="1" width="3" height="14" rx="1"></rect> <rect x="9" y="1" width="3" height="14" rx="1"></rect> </svg> </button>
Ok, let’s address the elephant in the room. Why do we have a separate button to play the video and a separate button to pause the video.
You might be thinking it’d be much easier to just change the icon between states. This works great for sighted users, but not for users who rely on a screen reader.
A few key points:
- aria-pressed - we’ll be updating this value with JavaScript to indicate if the button is pressed or not
- aria-label - will be read out loud by screen readers
- title - will display the text when a user hovers over the button, just like the title attribute does
For a more in-depth explanation, make sure to read Building Inclusive Toggle Buttons by Heydon Pickering.
Adding some style
Instead of jumping straight in, let’s progressively enhance the markup we’ve created.
First things first, let’s hide the buttons we just added if they aren’t needed:
.no-enhance .m-video__button { display: none; }
By default, I’ve added a no-enhance class to the html element. This means if the browser doesn’t have JavaScript turned on or the browser doesn’t support the features we need for the JavaScript to work (we’ll look at this later), then we’ll hide the buttons as they won’t do anything.
Styling our play/pause buttons
Ok, now we can start layering more styles on to get our buttons looking how we want them.
.a-button { background-color: #ffffff; border-radius: 100%; cursor: pointer; position: relative; transition: box-shadow 0.3s cubic-bezier(0.5, 0.61, 0.355, 1), opacity 0.3s cubic-bezier(0.5, 0.61, 0.355, 1); } .a-button:focus { box-shadow: 0 0 0 0.25rem rgba(255, 255, 255, 0.5); outline: none; } .a-button:hover { opacity: 0.75; } .a-button__icon { padding: 0.625rem; } .a-icon { fill: #000000; height: 2.1875rem; vertical-align: top; width: 2.1875rem; } @media screen and (min-width: 32em) { .a-icon { height: 2.5rem; width: 2.5rem; } }
Here’s the CSS we need to style up our play and pause buttons. Nothing too fancy here (if you need to support browsers further back then you can add in fallbacks for rem, inline SVG etc).
We won’t be covering that in this post, but if enough people want a solution that goes back to the prehistoric period, then I’ll update this post.
Styling our HTML5 video
We need a surprisingly little amount of CSS for our HTML5 video:
.m-video { background-color: #000000; position: relative; } .m-video__video { display: block; width: 100%; } .m-video__button { bottom: 0.9375rem; left: 0.9375rem; position: absolute; z-index: 20; } .m-video__button[aria-pressed="true"] { display: none; }
Most of this should be pretty self-explanatory. What I will explain, however, is this block of code:
.m-video__button[aria-pressed="true"] { display: none; }
What we’re doing here is hiding the play/pause button that has already been pressed.
So if the user clicks the play button the aria-pressed value will get set to true and the play button will get hidden (at least it will, when we have the JavaScript set up).
We’re on our way to having a great replacement for animated GIFs. So let’s carry on.
Hooking up our JavaScript
By now we have already created our autoplaying HTML5 video and added our play/pause buttons. The only issue is, our buttons don’t actually do anything yet.
So let’s add in some JavaScript to get this working. We’ll be making use of ES6 classes and other features in our demo.
First of all, let’s add in some script to check if the browser supports the features we need and if it does we’ll execute our video JavaScript:
/* Check if the users browser supports these features */ const enhance = "querySelector" in document && "addEventListener" in window && "classList" in document.documentElement; /* If the users browser browser supports the features we need, remove the no-enhance class from the html element and execute our video JS */ if(enhance) { document.documentElement.classList.remove("no-enhance"); /* Find all video molecules and instantiate a new instance of the Video class */ const videos = document.querySelectorAll(".js-video"); Array.from(videos).forEach((video) => { const videoEl = new Video(video); }); }
Now that we have that setup, we can create our video JavaScript:
class Video { constructor(video) { this.videoContainer = video; this.video = this.videoContainer.querySelector(".js-video-video"); this.playButton = this.videoContainer.querySelector(".js-video-play-button"); this.pauseButton = this.videoContainer.querySelector(".js-video-pause-button"); this.prefersReducedMotion(); this.addEventListeners(); } prefersReducedMotion() { /* If the users browser supports reduced motion and the user has set it to true, remove the autoplay attribute from the video and pause it */ if(matchMedia("(prefers-reduced-motion)").matches) { this.video.removeAttribute("autoplay"); this.pauseVideo(); } } addEventListeners() { this.playButton.addEventListener("click", () => { this.playVideo(); /* Focus the pause button so keyboard users can immediately pause the video without having to tab away and back again */ this.pauseButton.focus(); }); this.pauseButton.addEventListener("click", () => { this.pauseVideo(); /* Focus the play button so keyboard users can immediately play the video without having to tab away and back again */ this.playButton.focus(); }); } playVideo() { this.video.play(); /* Set the play button as pressed so it’s hidden and the pause button is displayed instead */ this.playButton.setAttribute("aria-pressed", "true"); this.pauseButton.setAttribute("aria-pressed", "false"); } pauseVideo() { this.video.pause(); /* Set the pause button as pressed so it’s hidden and the play button is displayed instead */ this.playButton.setAttribute("aria-pressed", "false"); this.pauseButton.setAttribute("aria-pressed", "true"); } }
I’ve added comments to the JavaScript so it should be pretty clear what’s going on.
That being said, I will expand on the prefersReducedMotion() method.
What is prefers reduced motion?
If this is the first time you’ve heard of the prefers-reduced-motion query; Val Head sums it up perfectly, in her post: The reduced motion query at a glance .
Essentially, we can tone down or remove motion that may be harmful to those with vestibular disorders or motion-sensitivities.
Currently, this feature is only supported in Safari, but will hopefully be adopted by other browsers sometime soon.
Mac: how to turn on reduced motion
If you have a mac you can turn on reduced motion by following these steps:
- Open System Preferences
- Click on Accessibility
- Click on Display
- Check the Reduce Motion checkbox
iOS: how to turn on reduced motion
If you have an iOS device (iPhone/iPad), you can turn on reduced motion by following these steps:
- Open Settings
- Tap on General
- Tap on Accessibility
- Tap on Reduce Motion
- Toggle on Reduce Motion
There you have it, an inclusive, progressively enhanced replacement for animated GIFs using HTML5 video.
If you’ve enjoyed this post I’d really appreciate it if you shared it around online. Equally, if you have any questions don’t hesitate to drop me a message on social media.
Videojs.com
Video.js blog.

Autoplay Best Practices with Video.js
- Never assume autoplay will work.
- Using the muted attribute/option will improve the chances that autoplay will succeed.
- Prefer programmatic autoplay via the player.play() method, avoiding the autoplay attribute/option.
Introduction
There has been a lot of chatter about autoplay lately and we wanted to take a few minutes to share some best practices when using autoplay with Video.js.
This post is meant to be a source of information - not an editorial or positional statement on autoplaying video. A lot of users hate autoplay because it's annoying or it consumes precious bandwidth. And a lot of publishers providing content free of charge rely on autoplay for the preroll ads that finance their businesses. Browser vendors (and open source library authors) have to weigh these concerns based on the interests of their users, publishers across the web, and their own business. And users have to choose the browser that best reflects their preferences.
This post is not about ways to circumvent autoplay policies. That's not possible and no one should waste their time attempting it. We think it's uncontroversial to say that actively circumventing the browser's built-in user experience or user preferences is harmful.
Autoplay Policies in the Big Browsers
I'm not going to go into exhaustive detail on each browser's specific algorithms because they are subject to change and it would defeat the core point of this post: never assume autoplay will work.
As of Safari 11, which shipped in September 2017, Apple has updated their autoplay policies to prevent autoplay with sound on most websites.
Back in September 2017, Google announced that Chrome's autoplay policy would change in April 2018 with Chrome 66 , subject to a series of rules you can read about in the linked article.
With Firefox, Mozilla has taken the position of not having a firm autoplay policy for the time being. That may change in the future. That said, Firefox today does allow users to disable autoplay with a few configuration changes.
IE11 and Edge
Microsoft's browsers have no specific/known autoplay policy - at the time of writing, autoplay just works in IE11 and Edge.
Refresher on How to Request Autoplay in Video.js
Note how the title of this section uses the word "request." That's intentional because it should be thought of as a request. Remember, never assume autoplay will work.
One of Video.js' strengths is that it is designed around the native <video> element interface; so, it will defer to the underlying playback technology (in most cases, HTML5 video) and the browser. In other words, if the browser allows it, Video.js allows it .
There are two ways to enable autoplay when using Video.js:
Use the autoplay attribute on either the <video> element or the new <video-js> element.
Use the autoplay option:
Recommended Practices
By default, you can defer to the default behavior in Video.js. If autoplay succeeds, the Video.js player will begin playing. If autoplay fails, the Video.js player will behave as if autoplay were off - i.e. it will display the "big play button" component over the poster image (or a black box).
If that works for you, your job is done!
TIP: If you want to use autoplay and improve the chances of it working, use the muted attribute (or the muted option, with Video.js).
Beyond that, there are some general practices that may be useful when dealing with autoplay: detecting whether autoplay is supported at all, or detecting whether autoplay succeeds or fails.
Detecting Autoplay Support
Similar to detecting successful or failed autoplay requests, it may be useful to perform autoplay feature detection on the browser before creating a player at all. In these cases, the can-autoplay library is the best solution. It provides a similar Promise -based API to the native player.play() method:
Programmatic Autoplay and Success/Failure Detection
For those who care whether or not an autoplay request was successful, both Google and Apple have recommended the same practice for detecting autoplay success or rejection: listen to the Promise returned by the player.play() method (in browsers that support it) to determine if autoplay was successful or not.
This can be coupled with the autoplay attribute/option or performed programmatically by calling player.play() , but we recommend avoiding the autoplay attribute/option altogether and requesting autoplay programmatically :
NOTE: It's important to understand that using this approach, the Video.js player.autoplay() method will return undefined or false . If you expect your users or integrators to rely on this method, consider the following section.
Programmatic Autoplay with the autoplay Attribute/Option
When building a player for others to use, you may not always have control over whether your users include the autoplay attribute/option on their instance of your player. Thankfully, combining this with programmatic autoplay doesn't seem to have a significant effect on the behavior of playback.
Based on our experimentation, even if the browser handles the actual autoplay operation, calling player.play() after it's begun playing (or failed to play) does not seem to cause current browsers to trigger an extra "play" event in either Chrome or Firefox. It does seem that Safari 11.1 triggers "playing" and "pause" events each time player.play() is called and autoplay fails.
Still, if you have full control, we recommend avoiding the autoplay attribute/option altogether and requesting autoplay programmatically .
NOTE: Even if using the autoplay attribute/option with programmatic autoplay, the player.autoplay() method will return undefined until the player is ready.
Example Use-Case
At Brightcove, one thing we've done to improve the user experience of our Video.js-based player is to hide the "big play button" for players requesting autoplay until the autoplay either succeeds or fails. This prevents a periodic situation where the "big play button" flashes on the player for a moment before autoplay kicks in.
While our actual implementation has more complexity due to specific circumstances with our player, the gist of it is the same as this functional JSBin demonstration.
There may be decision points in your code related to whether autoplay succeeds or not; however, the practical reality is that all the paragraphs before this boil down to a single, essential concept:
Never assume that your request to autoplay will succeed.
Keep that in mind and you're golden. Even if the browsers stop allowing autoplay entirely, our hope is that recommending this approach will be somewhat future-proof.

DEV Community

Posted on Oct 17, 2019
How to fix autoplay html5 video on iOS?
If you have used Javascript to play an html5 video, you may encounter some errors, including this error:
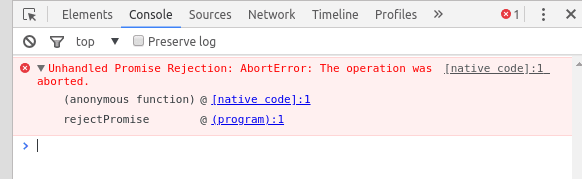
or permission errors.
The reason is that browsers in recent years changed their policies to prevent autoplay video because they think users don't want it (that's correct in most cases).
What we can do to solve this issue?
- Add autoplay , muted and playsinline attributes to your <video> element.
- If you need sound, you should put an unmute button beside the video, then in onClick callback, get video element and set muted = false by Javascript.
Pro Tip! If you have multiple videos on your page and only a single unmute button, then make sure to unmute all videos in the click callback of that button, otherwise, iOS won't let you unmute video before playing it on demand.
That's it, happy autoplaying!
Top comments (2)
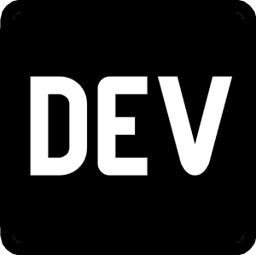
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Aug 18, 2020
What if I don't want the video to autoplay?

- Joined Sep 4, 2019
In that case just don't add autoplay attribute to the video tag. Adding autoplay="false" or autoplay="true" are equal and will autoplay the video.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse

Answer: Flutter geolocator package not retrieving location
Anurag Dhunna - Jun 20

Mastering Local Storage in Web Development
CodePassion - Jul 24

How I build simple Mac apps using Go
Jeff Lindsay - Jul 15

Hover Preview: A must have VsCode Extension for Webdevelopers 🔥
Paul - Jul 23
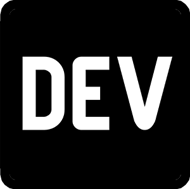
We're a place where coders share, stay up-to-date and grow their careers.
HTML References
Html <video> autoplay attribute.
❮ HTML <video> tag
A video that will automatically start playing:
Definition and Usage
The autoplay attribute is a boolean attribute.
When present, the video will automatically start playing.
Note: Chromium browsers do not allow autoplay in most cases. However, muted autoplay is always allowed.
Add muted after autoplay to let your video file start playing automatically (but muted).
Browser Support
The numbers in the table specify the first browser version that fully supports the attribute.

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.

HTML5 video autoplay on mobile revisited
There have been some changes since the last time I tested autoplay videos . Animated GIFs have become popular, because they work on mobile. A problem is that animated GIFs can become very large . A video is typically a lot smaller. That is why Apple and Google decided to allow autoplay in their mobile browsers. But only if videos are muted . On iOS you also have to set the playsinline attribute, because by default videos will play fullscreen.
To get autoplay use the following setup:
My tests show that the latest mobile browsers now support autoplay with the exception of MS Edge.
[update] Opera Mini on iOS 10 supports autoplay, but plays the video fullscreen. Other versions do not autoplay as one would expect from a proxy browser.
[update] Firefox on iOS does not support autoplay. This is very different from Firefox on Android that supports autoplay even with sound.
[update] On Samsung devices, the default browser is Samsung Internet . It is uses the same basis as Chrome, but it's not updated as frequently.
You can test for yourselves on my autoplay test pages:
Of course, you do not have to use autoplaying videos everywhere.
How to fix HTML video issues in iOS Safari
HTML videos in iOS Safari seem to have unique behaviour compared to their Chromium & Firefox counterparts. While working through this issue, I found a few solutions to some painful problems.
- My video expands when scrolling
Add the playsinline attribute to your video element.
- My background video pauses if pressed
Style the video element with user-select: none; to prevent the video from being paused when clicked or pressed.
- canplaythrough does not trigger
I’ve yet to solve this issue, but a helpful StackOverflow question about the audio element may have some hints.
This post is mostly to document my troubleshooting for the inevitable future problems I face, so if I get around to solving it, I’ll update this section with a code snippet.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
HTMLMediaElement: autoplay property
Baseline widely available.
This feature is well established and works across many devices and browser versions. It’s been available across browsers since July 2015 .
- See full compatibility
- Report feedback
The HTMLMediaElement.autoplay property reflects the autoplay HTML attribute, indicating whether playback should automatically begin as soon as enough media is available to do so without interruption.
A media element whose source is a MediaStream and whose autoplay property is true will begin playback when it becomes active (that is, when MediaStream.active becomes true ).
Note: Sites which automatically play audio (or videos with an audio track) can be an unpleasant experience for users, so it should be avoided when possible. If you must offer autoplay functionality, you should make it opt-in (requiring a user to specifically enable it). However, autoplay can be useful when creating media elements whose source will be set at a later time, under user control.
For a much more in-depth look at autoplay, autoplay blocking, and how to respond when autoplay is blocked by the user's browser, see our article Autoplay guide for media and Web Audio APIs .
A boolean value which is true if the media element will begin playback as soon as enough content has loaded to allow it to do so without interruption.
Note: Some browsers offer users the ability to override autoplay in order to prevent disruptive audio or video from playing without permission or in the background. Do not rely on autoplay actually starting playback and instead use play event.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- HTMLMediaElement : Interface used to define the HTMLMediaElement.autoplay property
- <audio> , <video>
How to Autoplay Videos in HTML?
This blog will start by explaining what HTML video autoplay is, its usage, and then the code snippet for implementing it. We will also cover how to use it in different scenarios and browser compatibility. Lastly, we will discuss how to fix HTML Video Autoplay not working error.
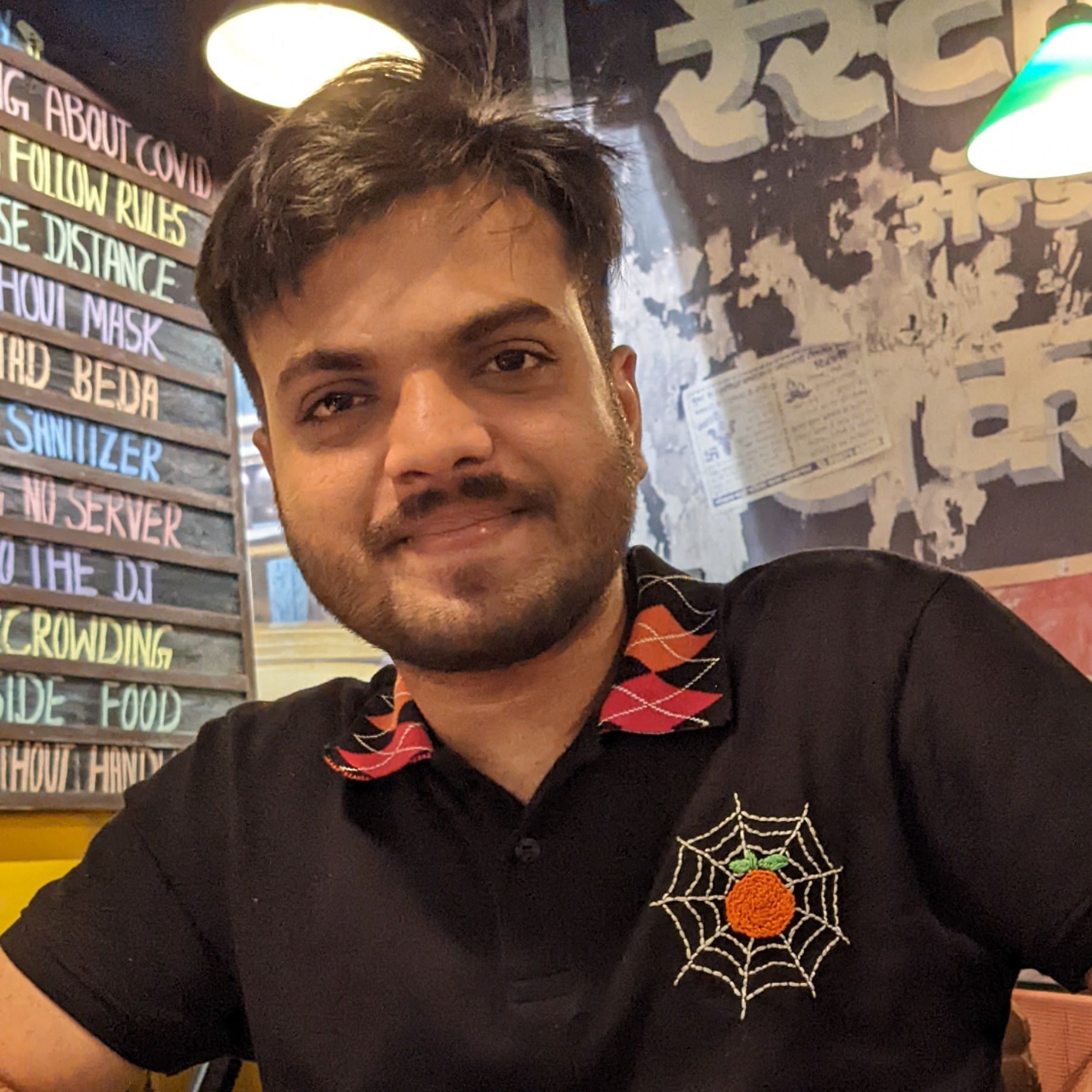
Shubham Hosalikar
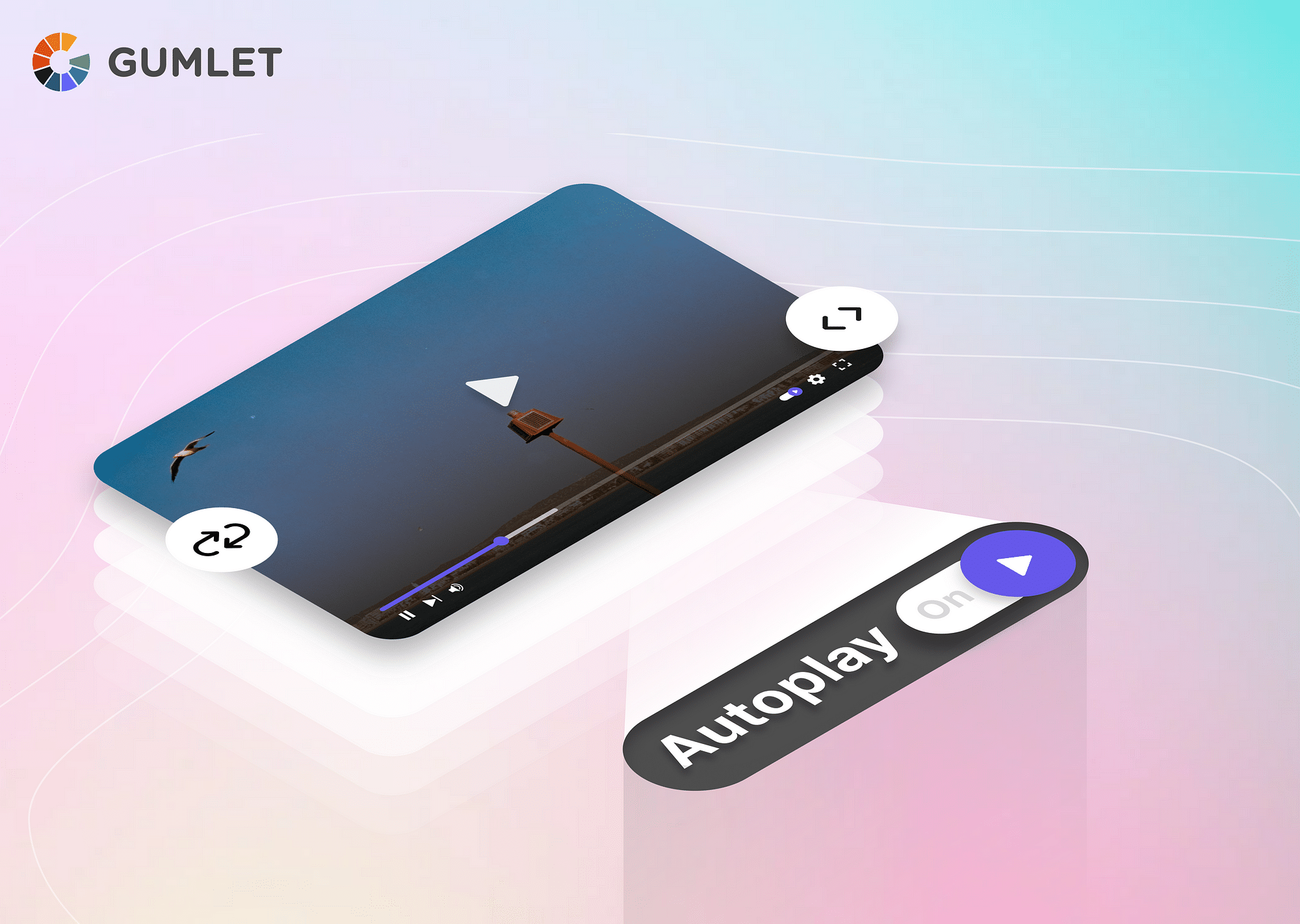
HTML Video Autoplay - What it means?
HTML Video Autoplay is a relatively new feature in HTML5 . It allows browsers to start playing a video automatically without requiring any trigger or interaction from the user. This can be achieved by adding the “autoplay” attribute, which is a boolean attribute, to the HTML video element. Although helpful in some avenues, this feature may often lead to negative user experiences. As such, most browsers and mobile devices have disabled the autoplay feature.
There is a workaround for this, however, as most browsers allow video autoplay under the following circumstances:
- The video is muted.
- The user has interacted with the site by clicking, tapping, or engaging with similar buttons.
Code Snippet for HTML Video Autoplay
To understand how the video autoplay attribute works, let us look at the code required to run this feature:
Let us understand the code's functionality by breaking it into different tags.
- <Video> Tag: The <video> tag is used initially to embed video on the relevant webpage.
- Autoplay Attribute: Autoplay is a boolean attribute that can be added to compel the browser to play the video automatically as soon as possible without waiting for the page to finish loading the data. With the autoplay attribute, the video will start playing as soon as the page loads and will be muted unless the user decides to unmute it manually.
- Controls Attribute: The control attribute allows users to gain control over how the video is played. If it's present, users can tinker with playback settings like volume, seeking, pause/resume option, etc.
- Width Attribute: The width attribute is present to set the dimensions and size of the video to be played on the webpage.
- <Source> Tag: The source tag, alongside src attributes, determine the file path of the video to be played.
- Type Attribute: The Type attribute specifies the video format , like MP4 , ogg, WebM , or any other format.
The video will start playing without any sound/volume if the video is playable. But if it is not playable, an error text will be sent to the viewers.
Browser Compatibility
Here is a list of the earliest browser versions that started supporting the video autoplay feature:
However, it’s imperative to understand that the autoplay feature will render differently on different web browsers.
For example, some browsers may require the video to be muted before the video starts playing automatically. In others, the users must engage with the page before automatic video playback occurs.
Different Cases for HTML Video Autoplay
In different cases, specific attributes can be used to change the functionality and behavior of the autoplay feature.
Muted Autoplay
Muted autoplay is a feature that allows browsers to support automatic video playback without sound. The user will have to enable the audio manually. With muted autoplay, browsers can provide appropriate visual appeal without disrupting the user experience.
Code Snippet:
Looped Autoplay
The looped attribute compels the browser to keep playing the video indefinitely in a loop unless the user pauses the video manually. This attribute is useful for a short promotional video that must be displayed on a particular web page.
Looped Autoplay Without Controls
Autoplay and loop attributes can be used together in case of silent animations on a website. In such a case, users cannot control the video playback.
Check if your video is optimized for instant start times on auto-play
Styling video autoplay with css.
To increase the aesthetic appeal of the video, different CSS elements can be incorporated to alter the video's dimensions and make other adjustments like adding backdrop colors and blurring effects.
Autoplay YouTube Video with iFrame Tag in HTML
In the case of YouTube content, the video tag cannot be used as YouTube does not allow users to access its raw video files. So, you will have to work with a unique video ID that does not match the actual file. As such, the element must be used.
Video autoplay is a powerful tool for the following:
- Displaying Promotional Videos: Websites can use the autoplay feature to automatically display advertisements to capture the user’s attention and convey information about specific products and services.
- News & Media Outlets: Autoplay feature is widely used on media websites to display the latest news and information without requiring any prompt from the users.
- Educational Materials: Many ed-tech websites use the video autoplay feature to start the automatic playback of important video content such as tutorials, lectures, and pre-recorded classes.
- Background Videos: Some websites use videos as backgrounds to increase the aesthetic appeal of their web pages. The video autoplay feature is often used to facilitate such background videos .
How to Fix HTML Video Autoplay Not Working?
In some cases, the video autoplay feature does not work. The most common root of this problem lies in the differing policies of different browsers. Some browsers only enable the autoplay feature when the volume is set to mute. So, ensure you have added the "muted" attribute next to the "autoplay" attribute.
Also, many developers make the mistake of using the autoplay attribute with the following syntax:
Despite being a boolean attribute, this syntax will only work when the video is set to mute or has no original audio of its own.
This is the primary fix that helped this problem for most users. However, if you're using iOS Safari, the syntaxes mentioned above won't work. You will have to add another attribute called 'playsinline'.
Here's how:
Note: It's generally recommended to use the HTML video autoplay instead of JavaScript, as the script could lead to displaying fallback content in case of errors.
In conclusion, auto-playing videos can enhance the user experience on your website, especially if you have a lot of multimedia content to share. However, it's important to remember that auto-playing videos can also be distracting and annoying if not implemented correctly. By following the steps outlined in this blog, you can easily add autoplay functionality to your videos in HTML. Remember always to provide a way for users to control the video playback and to consider the impact on page load times and user data usage.
Frequently Asked Questions (FAQs)
1. How to disable video autoplay in HTML?
The only way to disable the video autoplay in HTML is to remove the attribute altogether. The command autoplay=”false” won’t work, and the video will continue to be displayed automatically unless the attribute is completely removed from the code.
2. How to autoplay video with sound in HTML?
You can autoplay a video with sound in HTML by using the “Autoplay” attribute without using the “muted” attribute inside the <video> tag. However, many browsers do not support the automatic playback of unmuted videos.
3. Why is HTML video autoplay not working on mobile?
Mobile devices have generally disabled the use of video autoplay to prevent unwanted data usage and optimize user experience by preventing interruptions.
4. How do I autoplay a video in Chrome HTML?
You can easily autoplay a video in Chrome HTML by including the “autoplay muted” attribute in the <video> tag.
Similar readings
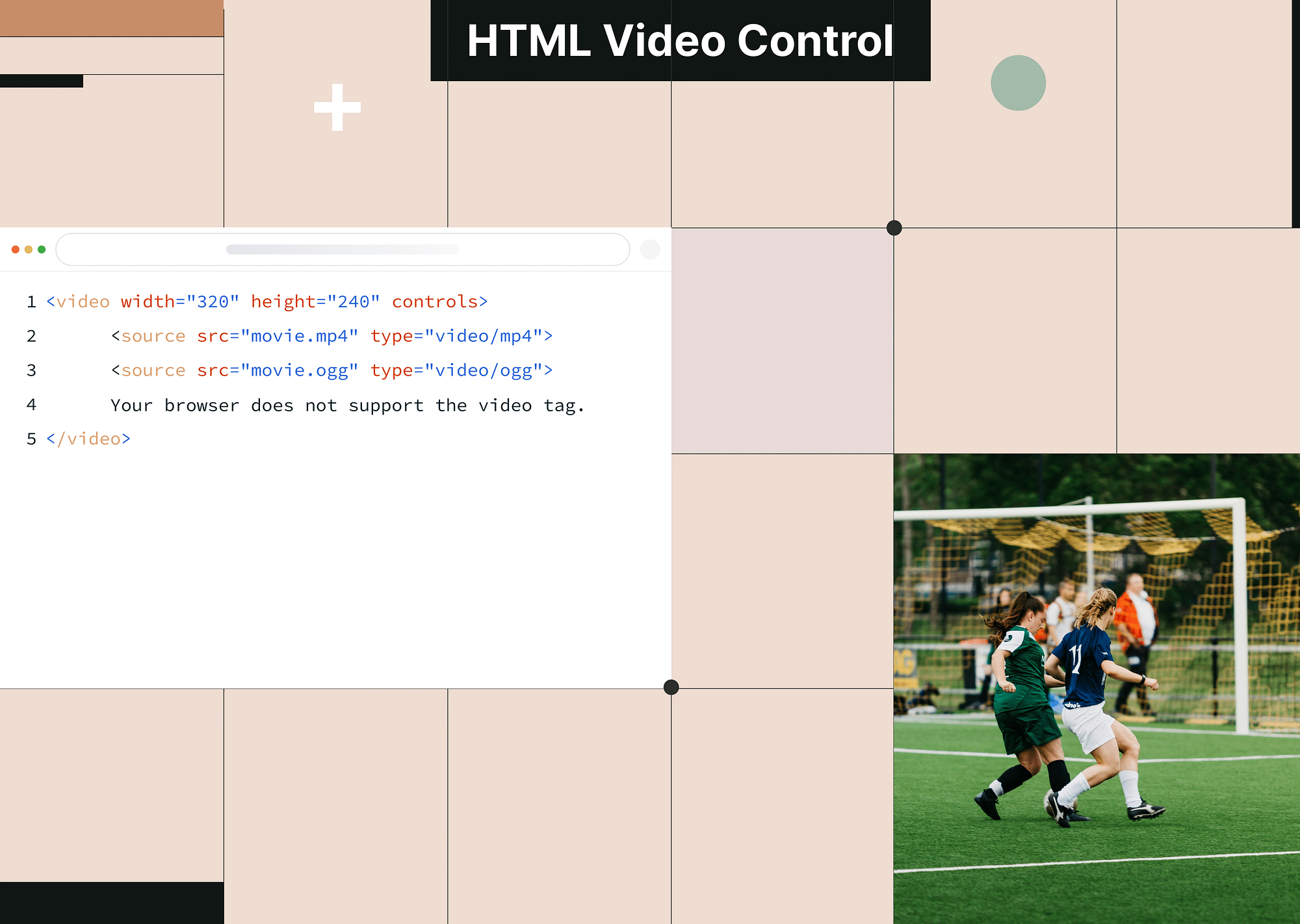
Images or Videos Loading Slow?
We compress them so your users don't need to wait.
Images or Videos Loading Slow? We compress them so your users don't need to wait. Try Gumlet →
Everything You Need to Know About HTML Video Autoplay
Judicial use is the secret sauce to HTML video autoplay.
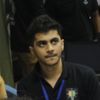
Read more posts by this author.
Autoplay is a common feature associated with web video. It allows multimedia content to begin playing automatically on page load without user interaction. For scenarios where immediate playback is beneficial (think background videos, or product demonstrations), the autoplay attribute ensures a smooth and user-friendly experience – your users don’t need to interrupt their browsing of a product to click a "play" button somewhere off to the side.
In this blog post, we'll delve into the topic of autoplay, exploring how this feature has revolutionized online content delivery, the pitfalls to avoid, and pairing it with ImageKit to make autoplay an asset (and not an annoyance!) for your website.
HTML5 Video and the autoplay attribute
In the past, to achieve such automatic video playback, developers had to use JavaScript to manipulate the video player. With HTML5, you can simply set the <video> element’s autoplay attribute (a boolean value) to specify that the video should start playing on page load, without the user specifically requesting playback.
This is a streamlined API that eliminates the need for JavaScript, complex embedding techniques, or third-party plugins, making it easier than ever to add video content to your website.
We’ve covered the specifics of HTML5 video here , so read up on that as a refresher. This post is going to focus entirely on the technical aspects of autoplay.
The basic HTML5 structure for an auto-playing video looks like this:
What happens when you set the autoplay property to true? Now, the following series of checks will take place:
- “Is the page allowed to use autoplay ?” – This might be unintuitive, but simply including the autoplay attribute might not be enough, as most browsers have their own set of conditions to check if autoplay can be allowed. These include checking if the media has an audio track, if the user has interacted with the page yet, etc. We'll talk more about these checks in a later section.
- “Was the <video> element created during page load?” – As in, not inserted programmatically later. Again, this makes sense as a guardrail against malicious intent.
- “Has a large enough chunk of the video been downloaded to begin and continue playback?” – This doesn’t mean the entire video must be downloaded before it can begin, just the minimum chunk needed to play something , and then download the rest as it plays through to the end. Notably, this check does not take into account network interruptions or unexpected bandwidth changes in the middle of playback.
As long as all three are true, the video will begin to autoplay.
Fleshing out our snippet with CSS and the controls attribute to add basic video player controls, here’s what it would look like in action, on a real webpage. You can check out the full code at this CodeSandBox here.
What are the benefits of HTML video autoplay?
HTML video autoplay offers several benefits when used thoughtfully. Some of these include:
- Boosting Engagement with Content: Autoplay immediately draws the user's attention to a video when they land on a webpage, perfect for showcasing engaging, new, or featured content. For example, Netflix autoplays trailers for hot and happening movies and shows as soon as you arrive. Users get a taste of the content this way and continue watching, consider subscribing, or consider staying subscribed.
- Reducing Bounce Rates with Seamless Navigation : Autoplay allows users to preview video content without having to manually click "play." YouTube, for example, often auto-plays related videos right after you finish watching one. This keeps the user invested with a steady stream of multimedia content, and when users encounter more videos that match their interests, they are more likely to stay on the website for longer periods, reducing bounce rates.
- Promotions and Storytelling: On Amazon, you’ll often see bite-sized autoplaying videos as banners to announce limited-time discounts or special offers. Conversely, a website for a non-profit or charity might autoplay a video on their landing page that showcases testimonials or the impact of their work, invoking emotions, establishing trust, and encouraging support.
- Product Demos and Tutorials: E-commerce websites employ autoplay to showcase product demos or tutorials. As users navigate to a product page, an explainer video can begin showcasing the item in use, highlighting the product's features and benefits. This helps users make informed purchase decisions.
- Design and Aesthetics: Autoplaying videos can run in silent background loops that add a dynamic and aesthetic element to your website. Consider a fashion brand's website with a subtle, looping animation of models walking down the runway, creating an aesthetic consistent with the brand. Or, autoplaying videos integrated into parallax scrolling, creating an immersive effect as users scroll down the page.
While autoplaying videos offer several advantages, it is critical to tread carefully with their implementation . If overused, or not correctly implemented, autoplay can actively harm the user experience (and accessibility) instead of enhancing it, and become disruptive and annoying. On mobile devices, misuse of autoplaying videos is even more intrusive due to limited screen real estate.
Moreover, there’s the issue of unoptimized, full-quality videos on autoplay that burn through data and battery, leading to slow loading times and lower PageSpeed Rankings.
To prevent this, consider using autoplay only in specific situations - where it adds value, such as for video previews, product showcases, or promotions. Judicial use is the secret sauce to autoplay.
Browser compatibility for HTML5 Autoplay
Most modern web browsers support the HTML video autoplay attribute. However, the behavior of autoplay may vary depending on the browser, and you need to meet certain conditions for it to work consistently.
First of all, here’s the current state of popular browser compatibility for the HTML5 autoplay attribute.
Data: HTML element: video: autoplay | Can I use... Support tables for HTML5, CSS3, etc
Secondly, it’s imperative to note that autoplay policies have evolved to enhance user experience and prevent intrusive or data-consuming behavior. Autoplay policies are different for each browser.
- User Interaction: Autoplay with sound is permitted on domains where the user has previously interacted.
- Previous Media Playback: If the user has played a video with sound on an active tab before, autoplay with sound is allowed.
- Mobile Engagement: For mobile users, if they have added the site to their home screen or installed the site’s Progressive Web App (PWA), autoplay with sound is permitted.
- Media Engagement Index (MEI): Chrome uses a metric called the Media Engagement Index to determine if videos should autoplay with sound. The MEI calculates this based on various factors, including the history of the user's interactions with media on the site.
- Mozilla Firefox : Firefox has a stricter autoplay policy. It generally blocks autoplay unless the audio is muted (or has its volume set to 0), the user has interacted with the site or the video element before, or the site has been explicitly whitelisted by the user.
- Apple Safari/Webkit : Apple’s policy uses an Automatic Inference Engine to block media elements with sound from auto-playing by default on most websites, but it gives granular control to users regarding whitelisting websites that are allowed to autoplay media content. Notably, Safari’s power saving mode prevents silent videos from autoplaying when hidden, in a background tab, or offscreen.
- Microsoft Edge : Starting with version 92, Edge follows a similar approach to Chrome, disabling autoplay with sound for all videos by default, while allowing some based on domains that users have interacted with previously.
- Opera : Opera's autoplay policy is the same as Chrome and Edge, being based on the same engine. It uses user interaction as a metric to determine whether a video should play with sound.
You should test on different browsers, and verify that your autoplaying videos work across them by taking account of the differences in browser behavior.
How to use HTML5 video autoplay with ImageKit?
ImageKit is an all-in-one, cloud-based, digital asset management solution that can perform real-time video (and image) optimization and transformation via a unique URL-based API that makes it easier to adapt your autoplaying videos for various devices and platforms. Then, with ImageKit’s global CDN, you can be sure they’re served from as close as possible to your users, letting you create seamless and interactive video experiences without getting caught up in the details.
Real-time Video Adaptation
One of ImageKit's standout features is its developer-friendly URL-based API. The API allows you to adapt videos on the fly – resizing, cropping, trimming, adjusting their quality in real time ( and more ) to suit different devices, screen sizes, and bandwidths.
Unoptimized, full-sized videos are the worst offenders when it comes to bad implementations of autoplay. To fix this, you’d normally need to create different variations of the same video, for different devices. With ImageKit, though, you avoid all of that work. Starting with a single source video, you can crop and resize them to pixel-perfect dimensions for different devices, or even different social media platforms – 16:9 for desktop viewers, square 1:1 for your mobile audiences or Instagram/Facebook feeds, or any arbitrary dimension based on your needs.
Simply add on the transforms you want to your video’s public URL, as query or path params. For example, here’s how easy it is to resize a source 1080p video to a 300px square one.
https://ik.imagekit.io/YOUR_ACCOUNT_NAME/YOUR_VIDEO.mp4?tr=w-300,h-300
Need a specific aspect ratio? Use the aspect ratio transform . This is for a 4:3 aspect ratio with a width of 480px.
https://ik.imagekit.io/YOUR_ACCOUNT_NAME/YOUR_VIDEO.mp4?tr=ar-4-3,w-480
What if you have a longer video and you want a short, looping snippet (say, starting at the 5-second mark, and lasting 5 seconds) to be played in a banner? You could use the trim transform.
https://ik.imagekit.io/YOUR_ACCOUNT_NAME/promo-2023.mp4?tr=so-5,du-5
Where so is the start time and du the duration, in seconds.
In fact, you could chain however many transformations you want to apply, in order, just like this.
Now, you can use this URL as the source wherever you want it to autoplay on your website or app.
ImageKit’s Transformation API proves invaluable for ensuring that your autoplay videos can be tailor-made for different user scenarios, devices, and platforms – all dynamically, and all from a single source video.
Automatic Video Format Conversion and Optimization
ImageKit automatically makes sure the most appropriate format for a given source video is served to your users – no matter which device or platform they’re consuming it from. All you need to do is turn on Automatic Format Conversion in the ImageKit dashboard and use the public URL to the video wherever you want, as usual. No need to manually create any variants yourself.
ImageKit can also automatically optimize video files to create a good enough balance between file size and image quality. This is a non-destructive process, and your source video is always intact. Again, this is another dashboard toggle – turn on Automatic Quality Optimization , tune the quality factor as desired, and you’re good to go.
Personalization and Enhancement
ImageKit's video transformation capabilities extend beyond basic optimization. You can personalize your videos by applying image, text, and video overlays, subtitles, and watermarks in multiple layers. For example, you can add your company's logo as a watermark to your autoplay videos, enhancing branding and engagement. Additionally, you can apply transforms even to these overlays you apply, to further tune them however you need.
HTML video autoplay is a powerful tool for enhancing user engagement and storytelling on your website, but you need to employ it judiciously, considering user preferences and ensuring the content aligns with your website's purpose. Consider some best practices like:
- Mute by Default : Mute your Autoplay videos (via the muted property) by default to prevent audio from startling or annoying users. If you use the loop attribute, ensure the video content is short in addition to being muted by default.
- Use ImageKit : Use ImageKit to dynamically optimize, transcode, and transform your video files to best fit your viewers’ devices, and your own needs. This ensures your viewers get the best quality at the best possible file sizes, reducing loading times and data usage.
- Inform Users: Communicate the presence of autoplay video on your website via a clearly visible container and play button. Then, delay the autoplay feature slightly to give users time to orient themselves on the page.
- Browser Compatibility: Test autoplay behavior across different browsers, as some may have specific policies or limitations on autoplaying videos. Also, test different autoplay strategies to see what works best for your specific audience.
- Don’t Ignore Accessibility : Provide descriptive alt text for the autoplaying video and captions (using the <track> element). This benefits users with visual or hearing impairments who rely on screen readers/text-to-speech.
Combining these autoplay strategies effectively with ImageKit, you can provide an enriched and dynamic web experience for your visitors, all while ensuring that your videos are optimized for performance and quality, and personalized to meet your unique requirements.
Remember that the key to success with HTML5 video autoplay is judicious usage and a user-friendly and responsible implementation.
- Web Browser
- Google Chrome Browser
- Mozilla Firefox Browser
- Microsoft Edge Browser
- Apple Safari Browser
- Tor Browser
- Opera Browser
- DuckDuckGo Browser
- Brave Browser
How to disable or enable auto-play videos in Apple Safari Browser ?
Auto-play videos, although they may prove convenient, annoy us, mostly, while we search on the internet. The default Apple Web browser, Safari, gives users an option of either allowing a video to play as soon as it opens the page, or not. Though this is an excellent feature aimed at enhancing a user’s experience, not every person appreciates this The next part of the paper will focus on why one would activate or deactivate auto-playing videos in Safari, as well as how these activities can be carried out.
What is Autoplay?
Autoplay is a website feature that allows video or audio to play without user intervention. The goal was to improve the user experience by making content more engaging and interactive. However as autoplay became more widely adopted by websites, users began reporting problems with the feature. These range from the inability to prevent unwanted sounds through sudden bursts of music, and even playing a video or song that was never intended for public viewing.
The main problem with autoplay is that it becomes a distraction. When you go to a website, for example, your intention may be to get certain information or perhaps do something. Autoplaying media turns your attention away and, even more annoying, it’s hard to stop them manually.
One problem with autoplay is that it can be overwhelming. The variety of audio and video available on media-heavy webpages can be tough. Even more so, if you’re using a mobile phone with limited battery power and data capacity.
Why Disable Autoplay?
You may need to disable autoplay for several different reasons. One problem is that autoplay can be annoying if you’re going to a web site full of videos and sound. Second, autoplay uses data and drains your battery life if you’re using a mobile device. Besides being distracting, it can also be annoying. If you’re trying to work in a quiet environment, this could get really on your nerves.
Disabling autoplay can also help protect your privacy. Some websites even use autoplay media to monitor your behavior and collect information about you. If you disable autoplay, websites can’t hoard too much data about yourself.
As a whole, autoplay can be helpful in some situations and it can also become an irritation. If autoplay is getting in your way, though, you might want to kill the feature at its source-in your browser.
Benefits of Enabling/Disabling the Feature:
Benefits of enabling auto-play:.
- Seamless Experience: It is a crucial element as well since if one wants to watch such videos seamlessly, auto-play is needed in which the video commences playing upon loading of the page and without pressing on any button.
- Convenience: Users would rather have automatic playback such as some news sites and social networking pages.
Benefits of Disabling Auto-Play:
- Data Conservation: For instance, a video that auto-plays as it is being downloaded may not be playable for users with limited bands. So this implies that in order to cut down on data usage one needs to turn off auto-play.
- Reduced Distractions: Some of the users will see it as an obstruction that hinders them from picking a specified set of videos that are relevant to them.
Steps to Enable Auto-Play Videos in Safari:
Step 1: Open Safari Preferences
Go to safari, select “preferences” on the tiptop menu bar and press it.
Step 2: Navigate to Websites
Go to menu bar and click preferences, after that we go to websites tab.
Step 3: Auto-Play Settings
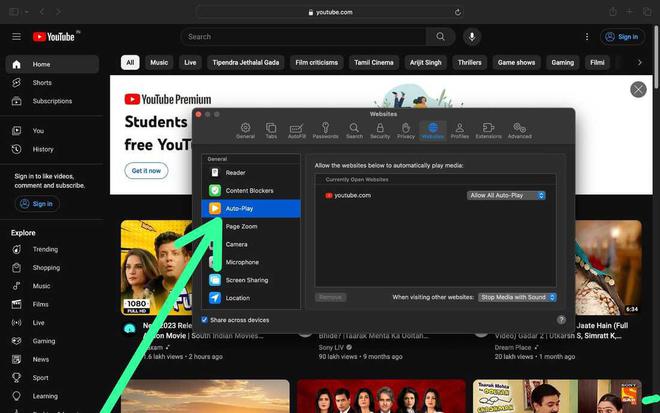
Go to the Left Side and click on Auto-Play.
Step 4: Enable Auto-Play
For the desired site, you will select the “allow all auto play” option.
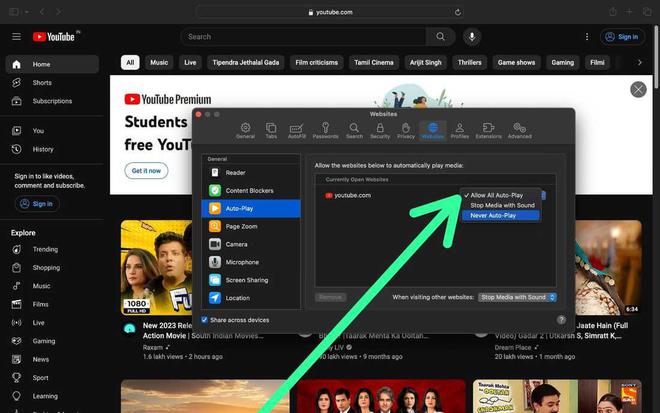
Step 5: Confirm Changes
To save your preferences, go to “OK” in the Preferences window.
Steps to Disable Auto-Play Videos in Safari:
From there head towards the menu bar and choose “Safari” then “Preferences”.
Click “webs” in the settings page.
On the list beside select Auto-Play.
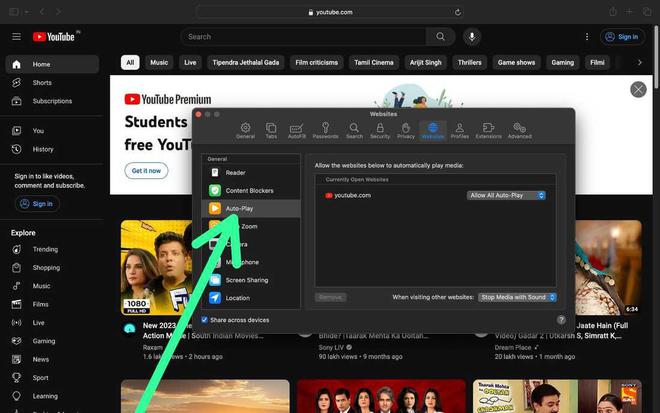
Step 4: Disable Auto-Play
Choose “shut off medial with audio” to turn off the automatic playing of video on the website.
-660.jpg)
Next, close the preferences window and you will have saved your settings.
Disabling Autoplay on Safari for iOS:
- Accessing Safari Settings on iOS:- Open your iOS device and bring up the “Settings” app from the home screen. Keep scrolling until you see Safari, then tap on it. On the right-hand side of your screen, there should now be a list of Safari settings.
- Stopping Autoplay in Videos and Audio:- Under the “Settings for Websites” section, tap on “Auto-Play.” You’ll now see three options: Allow All Auto-Play, Stop Media with Sound and Never Auto Play selections. To turn off autoplay completely, choose “Never Auto Play.” With this option selected no video or audio content will start playing automatically on any website.
- Troubleshooting Common Issues:- If you’ve followed the steps above but still experience autoplay, there are a few things you can try:
- Autoplay Still Active After Disabling:- If you still hear autoplay content after disabling it, clear your cache and cookies. However, these files sometimes interfere with Safari’s settings and cause unwelcome behavior.
- Reset Safari Autoplay Settings to Default:- If it’s still not working, you can reset Safari’s autoplay settings to defaults by going to “Safari” > Preferences>Web sites-AutoPlay and clicking on the Reset Default button in the lower right hand corner of window.
Conclusion:
Managing autoplay in Safari for personalizing browsing adventure. They enable people to toggle between two modes – “autopilot” and by-schedule at their convenience. Auto play videos can be enabled or disabled using these simple steps. Safari, travel great and manage it on your own.
Please Login to comment...
Similar reads.
- Geeks Premier League
- Web Browsers
- Apple Safari
- Geeks Premier League 2023
- SUMIF in Google Sheets with formula examples
- How to Get a Free SSL Certificate
- Best SSL Certificates Provider in India
- Elon Musk's xAI releases Grok-2 AI assistant
- Content Improvement League 2024: From Good To A Great Article
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
You can make a difference in the Apple Support Community!
When you sign up with your Apple ID , you can provide valuable feedback to other community members by upvoting helpful replies and User Tips .
Looks like no one’s replied in a while. To start the conversation again, simply ask a new question.
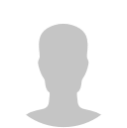
Safari doesn’t auto play videos
Some websites safari doesn’t auto play videos and other websites they run a little slow than in other browsers.
What do you recommend to do?
MacBook Pro 15″, macOS 13.0
Posted on Oct 6, 2022 12:58 PM
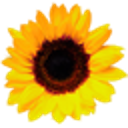
Posted on Oct 16, 2022 7:26 AM
It's possible the specific website you're attempting to view has settings that stop the video from automatically loading. You can reach out to the web developer to find out for sure.
You can customize settings for specific websites in Preferences in Safari. The steps below will guide you through this so specific websites load the way you'd like them to.

- Choose the options you want for the website (options vary by website):
- Use Reader when available: View webpage articles without ads or other distractions.
- Enable content blockers: Stop ads and other unwanted content from appearing.
- Page Zoom: Make text and images easier to see.
- Auto-Play: Choose whether video can play automatically on the website.
- Pop-up Windows: Choose whether the website can show pop-up windows.
- Camera: Choose whether the website can use your camera.
- Microphone: Choose whether the website can use your microphone.
- Screen Sharing: Choose whether the website can use your camera and microphone.
- Location: Choose whether the website can know your location."
This information is from Customize settings for each website in Safari on Mac - Apple Support .
Similar questions
- Video isn’t playing on websites on Safari I just moved to a new computer and my old computer used to play videos right away when I opened a webpage. It’s not doing it now. 1017 2
- Safari - why so many bugs ? (YouTube etc.) I wonder why Safari continues to have so many bugs ? (unlike most other browsers) For example YouTube: 1) You can't play videos in 4 or 8k 2) Every now and then the video does not start, you have to reload the page 3) Every now and then video stops playback, you need to reload. Many websites do not show correctly (in other browsers yes) Why is this happening ? 1711 13
- videos not loading AFL.com webpage videos won't load on safari but will on chrome 297 1
Loading page content
Page content loaded
Oct 16, 2022 7:26 AM in response to ferreirex
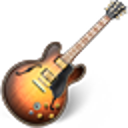
Oct 8, 2022 7:12 AM in response to ferreirex
Hey there ferreirex,
It sounds like you are having problems regarding autoplay and performance with some sites. You are able to change autoplay settings by following:
Stop autoplay videos in Safari on Mac
1. In the Safari app on your Mac, choose Safari > Settings for This Website.
You can also Control-click in the Smart Search field, then choose Settings for This Website.
2. Hold the pointer to the right of Auto-Play, then click the pop-up menu and choose an option:
* Allow All Auto-Play: Lets videos on this website play automatically.
It may also be helpful to refer to the steps linked below to address issues with specific site functionality.
If Safari on Mac doesn't open a webpage or isn’t working as expected
Have a good one!
Oct 16, 2022 6:36 AM in response to Chris_C1
Thanks but still have the same troubles, I have activated for all the videos auto play.
In the next link it is an exemple the video doesnt start the auto play
https://www.awwwards.com/inspiration/art-direction-and-photograpy-portfolio
There is any option in the General Settings for the Safari work as brand new instalation? (just trying to make this and a few more things work as I wish)
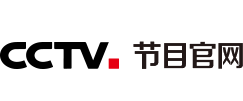
国防部例行记者会:第十一届北京香山论坛参会人员数量和层级再创新高。
- 视频简介 国防部例行记者会:第十一届北京香山论坛参会人员数量和层级再创新高。
中央广播电视总台 央视网 版权所有

IMAGES
VIDEO
COMMENTS
From Apple's documentation: Video elements that include <video autoplay> play automatically when the video loads in Safari on macOS and iOS, only if those elements also include the playsinline attribute. By default, autoplay executes only if the video doesn't contain an audio track, or if the video element includes the muted attribute.
Here is a simple solution to auto-play the video in IOS, I've already tried and it is perfectly working on IOS, Android, also on all the browsers on various platforms. simply use (data-wf-ignore) and (data-object-fit) Attributes for the video and data-wf-ignore for the source tag.. ... Autoplay video HTML on Safari iOS 14.2. Hot Network Questions
This video element is configured to include the user controls (typically play/pause, scrubbing through the video's timeline, volume control, and muting); also, since the muted attribute is included, and the playsinline attribute that is required for autoplay in Safari, the video will autoplay but with the audio muted. The user has the option ...
For example, autoplay video in interfaces where video playback is the purpose of the webpage, like a video-sharing or hosting website. Optimize Video Content for Safari To ensure that video content takes advantage of the hardware and software optimizations of your machine and Safari, get your video into low-power mode and use MP4 files instead ...
Fix HTML Video Autoplay Not Working April 12th, 2022. To optimise pages on this blog I recently replaced the animated GIFs with auto-playing videos. It required a couple tries to get it right, so here's how it works. ... However the video above still won't autoplay on iOS Safari, Safari requires us to add the playsinline attribute.
MP4: 836kb. Savings: 574%. That's a huge saving! Unfortunately, it does mean more work on our part to set up the markup needed to create an auto-playing HTML5 video. It's also worth noting, prior to iOS10 auto-playing HTML5 video wasn't possible on iOS devices.
One of Video.js' strengths is that it is designed around the native <video> element interface; so, it will defer to the underlying playback technology (in most cases, HTML5 video) and the browser. In other words, if the browser allows it, Video.js allows it. There are two ways to enable autoplay when using Video.js:
Add autoplay, muted and playsinline attributes to your <video> element. If you need sound, you should put an unmute button beside the video, then in onClick callback, get video element and set muted = false by Javascript. Pro Tip! If you have multiple videos on your page and only a single unmute button, then make sure to unmute all videos in ...
Modern browsers allow you to autoplay video if it : Only if things were simple! By now most of the browsers will be able to autoplay the video. Issue 1: React does not guarantee that muted ...
The autoplay attribute is a boolean attribute. When present, the video will automatically start playing. Note: Chromium browsers do not allow autoplay in most cases. However, muted autoplay is always allowed. Add muted after. autoplay to let your video file start playing automatically (but muted).
My tests show that the latest mobile browsers now support autoplay with the exception of MS Edge. [update] Opera Mini on iOS 10 supports autoplay, but plays the video fullscreen. Other versions do not autoplay as one would expect from a proxy browser. [update] Firefox on iOS does not support autoplay. This is very different from Firefox on ...
HTML videos in iOS Safari seem to have unique behaviour compared to their Chromium & Firefox counterparts. While working through this issue, I found a few solutions to some painful problems. Add the playsinline attribute to your video element. Style the video element with user-select: none; to prevent the video from being paused when clicked or ...
The HTMLMediaElement.autoplay property reflects the autoplay HTML attribute, indicating whether playback should automatically begin as soon as enough media is available to do so without interruption.. A media element whose source is a MediaStream and whose autoplay property is true will begin playback when it becomes active (that is, when MediaStream.active becomes true).
HTML Video Autoplay - What it means? HTML Video Autoplay is a relatively new feature in HTML5. It allows browsers to start playing a video automatically without requiring any trigger or interaction from the user. This can be achieved by adding the "autoplay" attribute, which is a boolean attribute, to the HTML video element.
Autoplay is a common feature associated with web video. It allows multimedia content to begin playing automatically on page load without user interaction. For scenarios where immediate playback is beneficial (think background videos, or product demonstrations), the autoplay attribute ensures a smooth and user-friendly experience - your users ...
Steps to Enable Auto-Play Videos in Safari: Step 1: Open Safari Preferences. Go to safari, select "preferences" on the tiptop menu bar and press it. Step 2: Navigate to Websites. Go to menu bar and click preferences, after that we go to websites tab. Step 3: Auto-Play Settings. Go to the Left Side and click on Auto-Play. Step 4: Enable Auto ...
A simple video element using an mp4, and I want it to autoplay when a person gets to the site, for both Safari versions, iOS/macOS. comments sorted by Best Top New Controversial Q&A Add a Comment
In the Safari app on your Mac, choose Safari > Settings for This Website. You can also Control-click in the Smart Search field, then choose Settings for This Website. 2. Hold the pointer to the right of Auto-Play, then click the pop-up menu and choose an option: * Allow All Auto-Play: Lets videos on this website play automatically.
Two male Chilean flamingos have successfully hatched an egg together in a rare first for a UK zoo. But how the flamingo dads got the egg is still unclear.
The U.S. is home to the majority of the world's data centers with just under 3,000. This concentration has brought up the value of the land where the data centers are built. Prices are soaring as ...
html. video. safari. autoplay. asked Nov 27, 2017 at 16:40. Josh. 1,377 3 14 24. 2. autoplay is becoming more supported as long as the video is muted, but if you un-mute without user intervention then the video will pause.
国防部例行记者会:第十一届北京香山论坛参会人员数量和层级再创新高。
Make sure you check browser permissions. If the site is not secure (http), then autoplay on websites will be disabled. To make changes you can click on the left side of the Address Bar of the browser and click Allow audio and video. edited Jul 27, 2021 at 15:45.
const myvideo = document.getElementById('myvideo'); myvideo.addEventListener('ended', (event) => { event.target.currentTime = 0; event.target.play(); });